Auto Complete trong Android
Nếu bạn muốn nhận các sự gợi ý khi bạn đang gõ vào trong trường text, thì bạn có thể thực hiện điều này thông qua AutoCompleteTextView. Nó cung cấp tự động các gợi ý khi người dùng đang gõ. Danh sách các gợi ý được hiển thị trong một dropdown menu từ đó người dùng có thể chọn một item để thay thế cho nội dung của phần mình vừa soạn.
Để sử dụng AutoCompleteTextView, đầu tiên bạn phải tạo một trường AutoCompleteTextView trong xml. Cú pháp như sau:
<AutoCompleteTextView android:id="@+id/autoCompleteTextView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="65dp" android:ems="10" >
Sau đó bạn phải lấy một tham chiếu của Textview này trong Java. Cú pháp như sau:
private AutoCompleteTextView actv; actv = (AutoCompleteTextView) findViewById(R.id.autoCompleteTextView1);
Việc tiếp theo bạn cần làm là xác định danh sách các mục gợi ý để được hiển thị. Bạn có thể xác định các mục này ở dạng một mảng chuỗi trong Java hoặc trong strings.xml. Cú pháp như sau:
String[] countries = getResources().getStringArray(R.array.list_of_countries); ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1,countries); actv.setAdapter(adapter);
Lớp ArrayAdapter chịu trách nhiệm hiển thị dữ liệu dạng danh sách trong hộp gợi ý của trường text. Phương thức setAdapter được sử dụng để thiết lập Adapter của AutoCompleteTextView. Ngoài các phương thức này, lớp AutoComplete còn cung cấp các đối tượng hữu ích khác, đó là:
Stt | Phương thức & Miêu tả |
---|---|
1 | getAdapter()
Phương thức này trả về một List adapter có thể lọc được được sử dụng cho Auto complete |
2 |
getCompletionHint()
Phương thức này trả về text gợi ý tùy ý được hiển thị ở dưới danh sách kết nối |
3 | getDropDownAnchor()
Phương thức này trả về view id mà danh sách dropdown được neo tới |
4 | getListSelection()
Phương thức này trả về ị trí của lựa chọn, nếu có một |
5 |
isPopupShowing()
This method indicates whether the popup menu is showing |
6 |
setText(CharSequence text, boolean filter)
Phương thức này thiết lập text mà ngoại trừ đó nó có thể vô hiệu hóa trình lọc/p> |
7 |
showDropDown()
Phương thức này hiển thị dropdown trên màn hình |
Ví dụ
Ứng dụng Android dưới đây minh họa cách sử dụng của lớp AutoCompleteTextView.
Sau đây là nội dung của src/MainActivity.java
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.content.Context; import android.media.AudioManager; import android.media.MediaPlayer; import android.media.MediaRecorder; import android.os.Bundle; import android.os.Environment; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.ArrayAdapter; import android.widget.AutoCompleteTextView; import android.widget.Button; import android.widget.EditText; import android.widget.ImageView; import android.widget.MultiAutoCompleteTextView; import android.widget.Toast; import java.io.IOException; public class MainActivity extends Activity { AutoCompleteTextView text; MultiAutoCompleteTextView text1; String[] languages={"Android ","java","IOS","SQL","JDBC","Web services"}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); text=(AutoCompleteTextView)findViewById(R.id.autoCompleteTextView1); text1=(MultiAutoCompleteTextView)findViewById(R.id.multiAutoCompleteTextView1); ArrayAdapter adapter = new ArrayAdapter(this,android.R.layout.simple_list_item_1,languages); text.setAdapter(adapter); text.setThreshold(1); text1.setAdapter(adapter); text1.setTokenizer(new MultiAutoCompleteTextView.CommaTokenizer()); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Sau đây là nội dung của activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Android Auto Complete" android:id="@+id/textView" android:textSize="30dp" android:layout_alignParentTop="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorialspoint" android:id="@+id/textView2" android:textColor="#ff3eff0f" android:textSize="35dp" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:src="@drawable/logo" android:layout_below="@+id/textView2" android:layout_alignLeft="@+id/textView2" android:layout_alignStart="@+id/textView2" android:layout_alignRight="@+id/textView2" android:layout_alignEnd="@+id/textView2" /> <AutoCompleteTextView android:id="@+id/autoCompleteTextView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:layout_below="@+id/imageView" android:layout_alignLeft="@+id/imageView" android:layout_alignStart="@+id/imageView" android:layout_marginTop="72dp" android:hint="AutoComplete TextView"> <requestFocus /> </AutoCompleteTextView> <MultiAutoCompleteTextView android:id="@+id/multiAutoCompleteTextView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:layout_below="@+id/autoCompleteTextView1" android:layout_alignLeft="@+id/autoCompleteTextView1" android:layout_alignStart="@+id/autoCompleteTextView1" android:hint="Multi Auto Complete " /> </RelativeLayout>
Sau đây là nội dung của Strings.xml
<resources> <string name="app_name">My Application</string> <string name="hello_world">Hello world!</string> <string name="action_settings">Settings</string> </resources>
Sau đây là nội dung của AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.autocomplete" android:versionCode="1" android:versionName="1.0" > <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.sairamkrishna.myapplication.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Cuối cùng, bạn chạy ứng dụng Android vừa tạo ở trên.
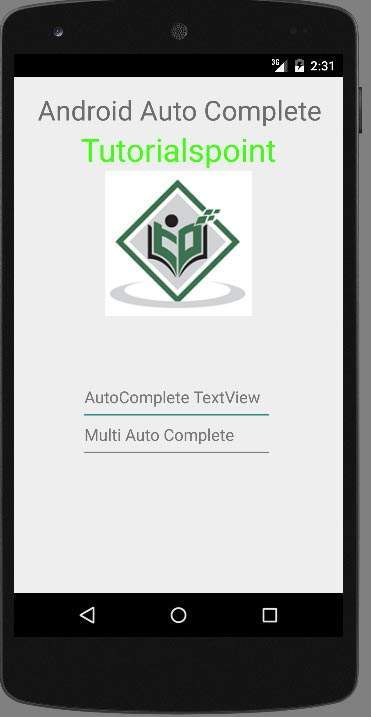
Bây giờ, soạn văn bản vào trong textview để thấy các gợi ý. Mình gõ một chữ cái làa, và nó chỉ cho mình các gợi ý.
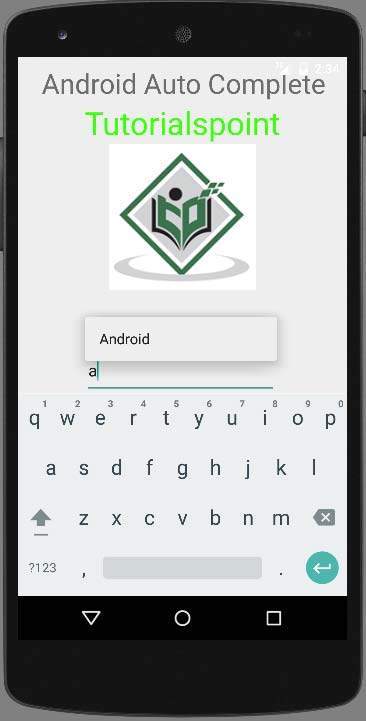
multiAutoCompleteTextView minh họa các gợi ý không chỉ cho một chữ cái mà cho cả một từ. Ví dụ, sau khi bạn viết từ đầu tiên, khi bạn bắt đầu viết từ thứ hai, nó sẽ hiển thị các gợi ý. Bạn có thể theo dõi hình sau:
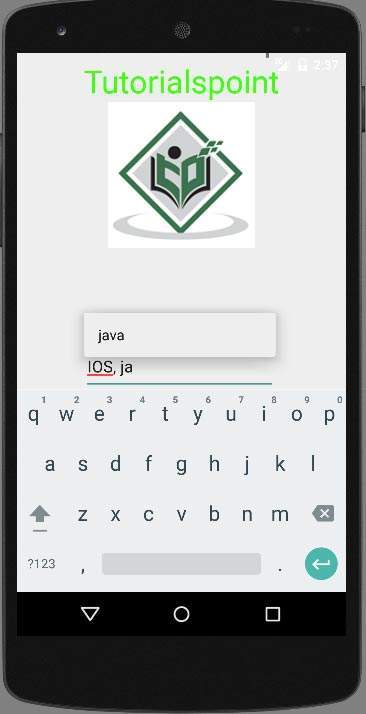
Đã có app VietJack trên điện thoại, giải bài tập SGK, SBT Soạn văn, Văn mẫu, Thi online, Bài giảng....miễn phí. Tải ngay ứng dụng trên Android và iOS.
Theo dõi chúng tôi miễn phí trên mạng xã hội facebook và youtube:Các bạn có thể mua thêm khóa học JAVA CORE ONLINE VÀ ỨNG DỤNG cực hay, giúp các bạn vượt qua các dự án trên trường và đi thực tập Java. Khóa học có giá chỉ 300K, nhằm ưu đãi, tạo điều kiện cho sinh viên cho thể mua khóa học.
Nội dung khóa học gồm 16 chuơng và 100 video cực hay, học trực tiếp tại https://www.udemy.com/tu-tin-di-lam-voi-kien-thuc-ve-java-core-toan-tap/ Bạn nào có nhu cầu mua, inbox trực tiếp a Tuyền, cựu sinh viên Bách Khoa K53, fb: https://www.facebook.com/tuyen.vietjack
Follow facebook cá nhân Nguyễn Thanh Tuyền https://www.facebook.com/tuyen.vietjack để tiếp tục theo dõi các loạt bài mới nhất về Java,C,C++,Javascript,HTML,Python,Database,Mobile.... mới nhất của chúng tôi.
Bài học Angular phổ biến tại vietjack.com: