Bluetooth trong Android
Bluetooth là một cách để truyền và nhận dữ liệu giữa hai thiết bị khác nhau. Android platform cung cấp hỗ trợ cho Bluetooth framework cho phép một thiết bị trao đổi dữ liệu với thiết bị Bluetooth khác.
Android cung cấp Bluetooth API để thực hiện các hoạt động:
Quét các thiết bị Bluetooth khác.
Lấy một danh sách các thiết bị có thể ghép cặp (Paired Device).
Kết nối với thiết bị khác thông qua dịch vụ dò tìm.
Android cung cấp lớp BluetoothAdapter để giao tiếp với Bluetooth. Tạo một đối tượng của lớp này bởi gọi phương thức static là getDefaultAdapter(). Cú pháp như sau:
private BluetoothAdapter BA; BA = BluetoothAdapter.getDefaultAdapter();
Để kích hoạt Bluetooth của thiết bị, gọi Intent với hằng ACTION_REQUEST_ENABLE. Cú pháp như sau:
Intent turnOn = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(turnOn, 0);
Ngoài hằng này, API còn cung cấp một số hằng khác để hỗ trợ các tác vụ khác nhau. Bảng dưới liệt kê các hằng này:
Stt | Constant & Miêu tả |
---|---|
1 | ACTION_REQUEST_DISCOVERABLE
Hằng này được sử dụng để bật trình dò tìm của Bluetooth |
2 |
ACTION_STATE_CHANGED
Hằng này thông báo rằng trạng thái Bluetooth đã bị thay đổi |
3 | ACTION_FOUND
Hằng này được sử dụng để nhận thông tin về mỗi thiết bị đã được dò tìm |
Khi bạn kích hoạt Bluetooth, bạn có thể lấy một danh sách các thiết bị có thể ghép cặp bằng cách gọi phương thức getBondedDevices(). Nó trả về một tập hợp các thiết bị Bluetooth. Cú pháp là:
private Set<BluetoothDevice>pairedDevices; pairedDevices = BA.getBondedDevices();
Ngoài các thiết bị có thể ghép đôi, API còn cung cấp một số phương thức khác để giúp bạn có nhiều điều khiển hơn thông qua Bluetooth.
Stt | Phương thức & Miêu tả |
---|---|
1 | enable()
Phương thức này kích hoạt Adapter nếu nó chưa được kích hoạt |
2 |
isEnabled()
Phương thức này trả về true nếu Adapter đã được kích hoạt |
3 |
disable()
Phương thức này vô hiệu hóa Adapter |
4 |
getName()
Phương thức này trả về tên của Bluetooth Adapter |
5 |
setName(String name)
Phương thức này thay đổi tên của Bluetooth |
6 |
getState()
Phương thức này trả về trạng thái hiện tại của Bluetooth Adapter |
7 |
startDiscovery()
Phương thức này bắt đầu tiến trình dò tìm của Bluetooth trong 120 s |
Ví
Ví dụ sau minh họa lớp BluetoothAdapter để thao tác Bluetooth và liệt kê các thiết bị có thể ghép đôi.
Để thử nghiệm ví dụ, bạn cần chạy trên một thiết bị thực sự.
Sau đây là nội dung của src/MainActivity.java
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.bluetooth.BluetoothAdapter; import android.bluetooth.BluetoothDevice; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.content.IntentFilter; import android.graphics.Color; import android.net.wifi.ScanResult; import android.net.wifi.WifiManager; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.EditText; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; import java.util.ArrayList; import java.util.List; import java.util.Set; public class MainActivity extends Activity { Button b1,b2,b3,b4; private BluetoothAdapter BA; private Set<BluetoothDevice>pairedDevices; ListView lv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); b1 = (Button) findViewById(R.id.button); b2=(Button)findViewById(R.id.button2); b3=(Button)findViewById(R.id.button3); b4=(Button)findViewById(R.id.button4); BA = BluetoothAdapter.getDefaultAdapter(); lv = (ListView)findViewById(R.id.listView); } public void on(View v){ if (!BA.isEnabled()) { Intent turnOn = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(turnOn, 0); Toast.makeText(getApplicationContext(),"Turned on",Toast.LENGTH_LONG).show(); } else { Toast.makeText(getApplicationContext(),"Already on", Toast.LENGTH_LONG).show(); } } public void off(View v){ BA.disable(); Toast.makeText(getApplicationContext(),"Turned off" ,Toast.LENGTH_LONG).show(); } public void visible(View v){ Intent getVisible = new Intent(BluetoothAdapter.ACTION_REQUEST_DISCOVERABLE); startActivityForResult(getVisible, 0); } public void list(View v){ pairedDevices = BA.getBondedDevices(); ArrayList list = new ArrayList(); for(BluetoothDevice bt : pairedDevices) list.add(bt.getName()); Toast.makeText(getApplicationContext(),"Showing Paired Devices",Toast.LENGTH_SHORT).show(); final ArrayAdapter adapter = new ArrayAdapter(this,android.R.layout.simple_list_item_1, list); lv.setAdapter(adapter); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Sau đây là nội dung của activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:transitionGroup="true"> <TextView android:text="Bluetooth Example" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textview" android:textSize="35dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials point" android:id="@+id/textView" android:layout_below="@+id/textview" android:layout_centerHorizontal="true" android:textColor="#ff7aff24" android:textSize="35dp" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:src="@drawable/abc" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:theme="@style/Base.TextAppearance.AppCompat" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Turn On" android:id="@+id/button" android:layout_below="@+id/imageView" android:layout_toStartOf="@+id/imageView" android:layout_toLeftOf="@+id/imageView" android:clickable="true" android:onClick="on" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Get visible" android:onClick="visible" android:id="@+id/button2" android:layout_alignBottom="@+id/button" android:layout_centerHorizontal="true" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="List devices" android:onClick="list" android:id="@+id/button3" android:layout_below="@+id/imageView" android:layout_toRightOf="@+id/imageView" android:layout_toEndOf="@+id/imageView" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="turn off" android:onClick="off" android:id="@+id/button4" android:layout_below="@+id/button" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <ListView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/listView" android:layout_alignParentBottom="true" android:layout_alignLeft="@+id/button" android:layout_alignStart="@+id/button" android:layout_below="@+id/textView2" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Paired devices:" android:id="@+id/textView2" android:textColor="#ff34ff06" android:textSize="25dp" android:layout_below="@+id/button4" android:layout_alignLeft="@+id/listView" android:layout_alignStart="@+id/listView" /> </RelativeLayout>
Sau đây là nội dung của Strings.xml
<resources> <string name="app_name">My Application</string> <string name="hello_world">Hello world!</string> <string name="action_settings">Settings</string> </resources>
Sau đây là nội dung của AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Cuối cùng, bạn chạy ứng dụng Android vừa tạo ở trên.
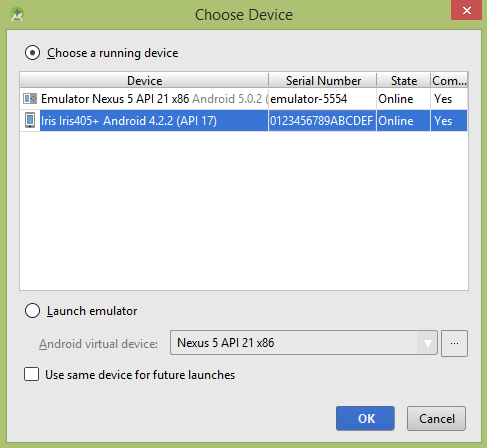
Bây giờ, chọn nút Turn On để bật Bluetooth. Nhưng khi bạn chọn nó, thì thì Bluetooth sẽ không được bật lên ngay, nó sẽ hỏi bạn về permission để kích hoạt Bluetooth.
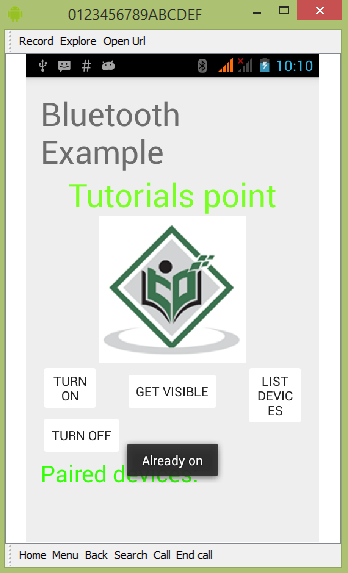
Bây giờ chọn nút GET VISIBLE để bật tính năng Visibility. Màn hình sau xuất hiện hỏi bạn về permission để bật dò tìm trong 120 s.
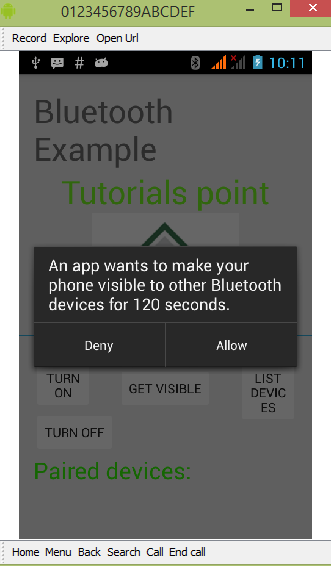
Chọn tùy chọn List Devices. Nó sẽ liệt kê các thiết bị có thể ghép đôi.
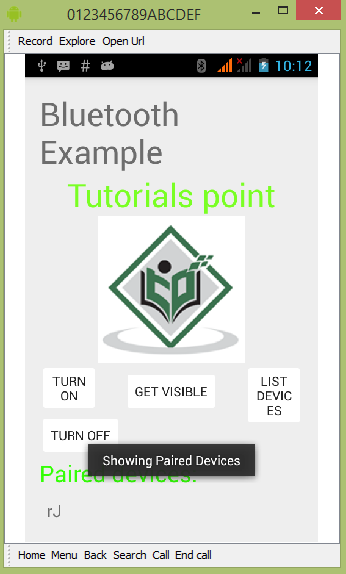
Chọn nút TURN OFF để tắt Bluetooth. Thông báo sau sẽ xuất hiện:
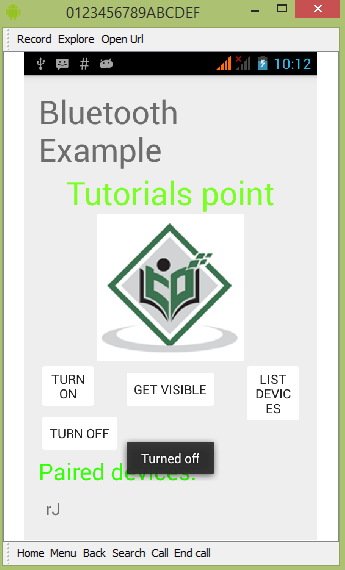
Đã có app VietJack trên điện thoại, giải bài tập SGK, SBT Soạn văn, Văn mẫu, Thi online, Bài giảng....miễn phí. Tải ngay ứng dụng trên Android và iOS.
Theo dõi chúng tôi miễn phí trên mạng xã hội facebook và youtube:Các bạn có thể mua thêm khóa học JAVA CORE ONLINE VÀ ỨNG DỤNG cực hay, giúp các bạn vượt qua các dự án trên trường và đi thực tập Java. Khóa học có giá chỉ 300K, nhằm ưu đãi, tạo điều kiện cho sinh viên cho thể mua khóa học.
Nội dung khóa học gồm 16 chuơng và 100 video cực hay, học trực tiếp tại https://www.udemy.com/tu-tin-di-lam-voi-kien-thuc-ve-java-core-toan-tap/ Bạn nào có nhu cầu mua, inbox trực tiếp a Tuyền, cựu sinh viên Bách Khoa K53, fb: https://www.facebook.com/tuyen.vietjack
Follow facebook cá nhân Nguyễn Thanh Tuyền https://www.facebook.com/tuyen.vietjack để tiếp tục theo dõi các loạt bài mới nhất về Java,C,C++,Javascript,HTML,Python,Database,Mobile.... mới nhất của chúng tôi.
Bài học Angular phổ biến tại vietjack.com: