Gesture trong Android
Android cung cấp nhiều kiểu sự kiện touch màn hình như những thao tác vuốt, kéo, thả, chạm, …. Chúng còn được biết với tên gọi là Gesture, và có thể nói đây là phần hay nhất của Smartphone.
Android cung cấp lớp GestureDetector để thu nhận các sự kiện di chuyển và nói cho chúng ta rằng các sự kiện này tương ứng với các Gesture hoặc không. Để sử dụng nó, bạn cần tạo một đối tượng GestureDetector và sau đó kế thừa lớp khác với GestureDetector.SimpleOnGestureListener để hoạt động như là một Listener và ghi đè một số phương thức. Cú pháp như sau: −
GestureDetector myG; myG = new GestureDetector(this,new Gesture()); class Gesture extends GestureDetector.SimpleOnGestureListener{ public boolean onSingleTapUp(MotionEvent ev) { } public void onLongPress(MotionEvent ev) { } public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) { } public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX, float velocityY) { } } }
Xử lý Pinch Gesture
Android cung cấp lớp ScaleGestureDetector để xử lý các Gesture như pinch, … Để sử dụng nó, bạn cần khởi tạo một đối tượng của lớp này. Cú pháp là: −
ScaleGestureDetector SGD; SGD = new ScaleGestureDetector(this,new ScaleListener());
Tham số đầu tiên là ngữ cảnh và tham số thứ hai là Event Listener. Chúng ta phải định nghĩa Event Listener và ghi đè một hàm là. OnTouchEvent . Cú pháp như sau: −
public boolean onTouchEvent(MotionEvent ev) { SGD.onTouchEvent(ev); return true; } private class ScaleListener extends ScaleGestureDetector.SimpleOnScaleGestureListener { @Override public boolean onScale(ScaleGestureDetector detector) { float scale = detector.getScaleFactor(); return true; } }
Ngoài Pinch Gesture, còn có một số phương thức khác để thông báo các sự kiện touch khác. Đó là: −
Stt | Phương thức & Miêu tả |
---|---|
1 |
getEventTime()
Phương thức này lấy thời gian sự kiện của sự kiện hiện tại đang được xử lý |
2 |
getFocusX()
Phương thức lấy tọa độ X của tiêu điểm Gesture hiện tại |
3 |
getFocusY()
Phương thức lấy tọa độ Y của tiêu điểm Gesture hiện tại |
4 |
getTimeDelta()
Phương thức này trả về sự khác nhau về thời gian giữa scale event trước và scale event sau |
5 |
isInProgress()
Phương thức này trả về true nếu một scale gesture là đang tiến hành |
6 |
onTouchEvent(MotionEvent event)
Phương thức này chấp nhận MotionEvents và gửi sự kiện khi thích hợp |
Ví dụ
Ví dụ sau minh họa sự sử dụng của lớp ScaleGestureDetector. Nó tạo một ứng dụng cơ bản để bạn phóng to và thu nhỏ thông qua Pinch Gesture.
Để thử nghiệm ví dụ này, bạn cần chạy trên thiết bị thật sự hoặc trong một Emulator với sự kiện touch màn hình được kích hoạt.
Sau đây là nội dung của Main Activity file đã được sửa đổi: src/MainActivity.java.
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.content.Intent; import android.content.IntentFilter; import android.graphics.Bitmap; import android.graphics.Matrix; import android.os.BatteryManager; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.MotionEvent; import android.view.ScaleGestureDetector; import android.view.SurfaceHolder; import android.view.SurfaceView; import android.view.View; import android.widget.ArrayAdapter; import android.widget.ImageView; import android.widget.TextView; import android.widget.Toast; import java.util.ArrayList; import java.util.Set; public class MainActivity extends Activity { private ImageView iv; private Matrix matrix = new Matrix(); private float scale = 1f; private ScaleGestureDetector SGD; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); iv=(ImageView)findViewById(R.id.imageView); SGD = new ScaleGestureDetector(this,new ScaleListener()); } public boolean onTouchEvent(MotionEvent ev) { SGD.onTouchEvent(ev); return true; } private class ScaleListener extends ScaleGestureDetector. SimpleOnScaleGestureListener { @Override public boolean onScale(ScaleGestureDetector detector) { scale *= detector.getScaleFactor(); scale = Math.max(0.1f, Math.min(scale, 5.0f)); matrix.setScale(scale, scale); iv.setImageMatrix(matrix); return true; } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Bạn sửa đổi nội dung của res/layout/activity_main.xml.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <TextView android:text="Gestures Example" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textview" android:textSize="35dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials point" android:id="@+id/textView" android:layout_below="@+id/textview" android:layout_centerHorizontal="true" android:textColor="#ff7aff24" android:textSize="35dp" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:src="@drawable/abc" android:scaleType="matrix" android:layout_below="@+id/textView" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentBottom="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout>
Và đây là nội dung của res/values/string.xml.
<resources> <string name="app_name>My Application</string> <string name="hello_world">Hello world!</string> <string name="action_settings">Settings</string> </resources>
Tiếp theo là nội dung của AndroidManifest.xml file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.gestures" android:versionCode="1" android:versionName="1.0" > <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.sairamkrishna.myapplicationMainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Chạy ứng dụng Android vừa tạo ở trên.
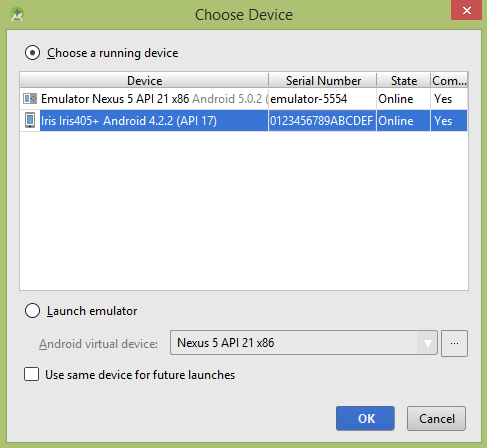
Chọn thiết bị mobile sẽ hiển thị màn hình mặc định là: −
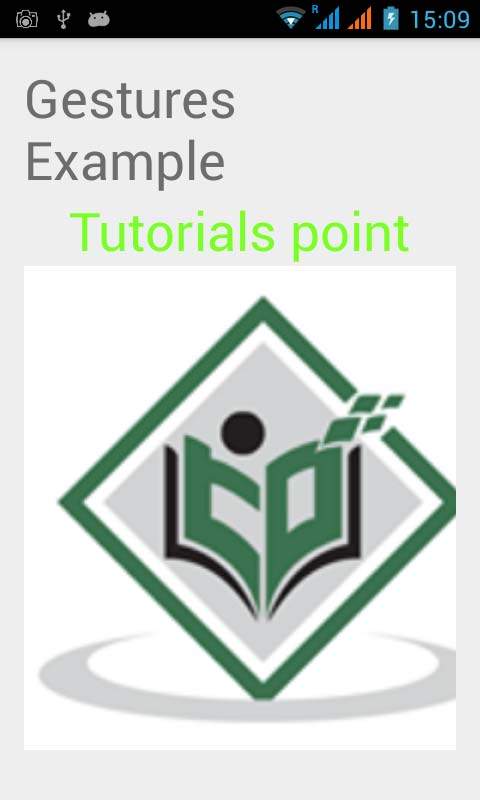
Bây giờ đặt hai ngón tay qua màn hình và sau đó di chuyển hai ngón tay sang hai bên, bạn sẽ thấy hình ảnh đang được phóng to hay thu nhỏ tùy theo cách bạn di chuyển. Hình dưới minh họa trường hợp phóng to. −
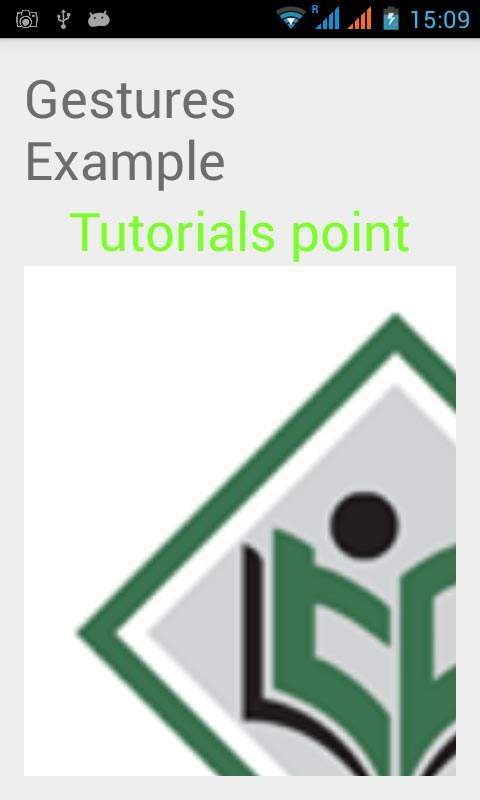
Hình sau minh họa trường hợp thu nhỏ. −
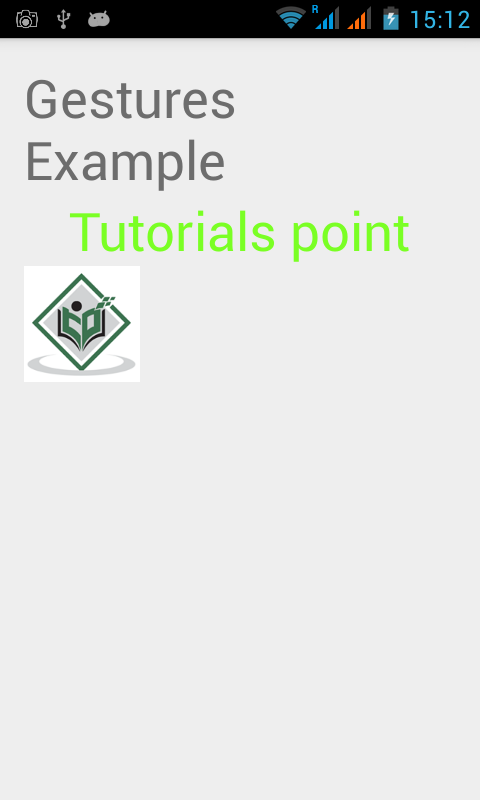
Đã có app VietJack trên điện thoại, giải bài tập SGK, SBT Soạn văn, Văn mẫu, Thi online, Bài giảng....miễn phí. Tải ngay ứng dụng trên Android và iOS.
Theo dõi chúng tôi miễn phí trên mạng xã hội facebook và youtube:Các bạn có thể mua thêm khóa học JAVA CORE ONLINE VÀ ỨNG DỤNG cực hay, giúp các bạn vượt qua các dự án trên trường và đi thực tập Java. Khóa học có giá chỉ 300K, nhằm ưu đãi, tạo điều kiện cho sinh viên cho thể mua khóa học.
Nội dung khóa học gồm 16 chuơng và 100 video cực hay, học trực tiếp tại https://www.udemy.com/tu-tin-di-lam-voi-kien-thuc-ve-java-core-toan-tap/ Bạn nào có nhu cầu mua, inbox trực tiếp a Tuyền, cựu sinh viên Bách Khoa K53, fb: https://www.facebook.com/tuyen.vietjack
Follow facebook cá nhân Nguyễn Thanh Tuyền https://www.facebook.com/tuyen.vietjack để tiếp tục theo dõi các loạt bài mới nhất về Java,C,C++,Javascript,HTML,Python,Database,Mobile.... mới nhất của chúng tôi.
Bài học Angular phổ biến tại vietjack.com: